In the Consumer Packaged Goods (CPG) industry, Distribution Management Systems (DMS) serve as the backbone of supply chain operations, ensuring seamless order fulfillment, effective inventory management, and robust retailer relationships.
As digital transformation accelerates, Artificial Intelligence (AI) and Machine Learning (ML) are redefining the potential of DMS, enhancing both efficiency and decision-making capabilities.
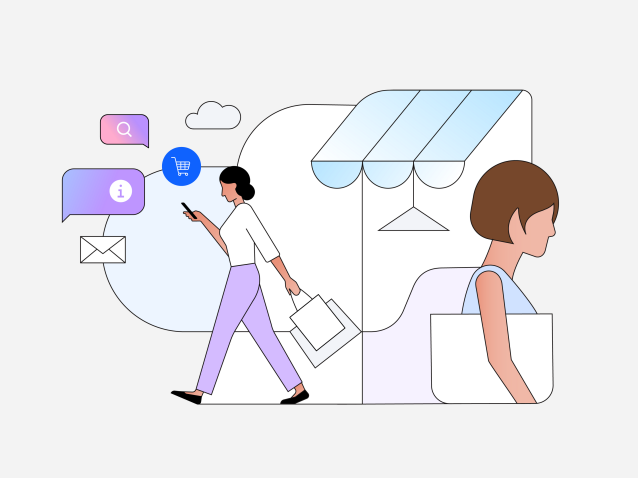
This article explores the pivotal role of AI/ML in modern DMS, key modules, and Python-based implementations that demonstrate these technologies in action.
The Role of AI/ML in DMS
AI and ML bring a revolutionary edge to Distribution Management Systems, enabling smarter operations, real-time insights, and predictive analytics. Below are key areas where these technologies are making a significant impact:
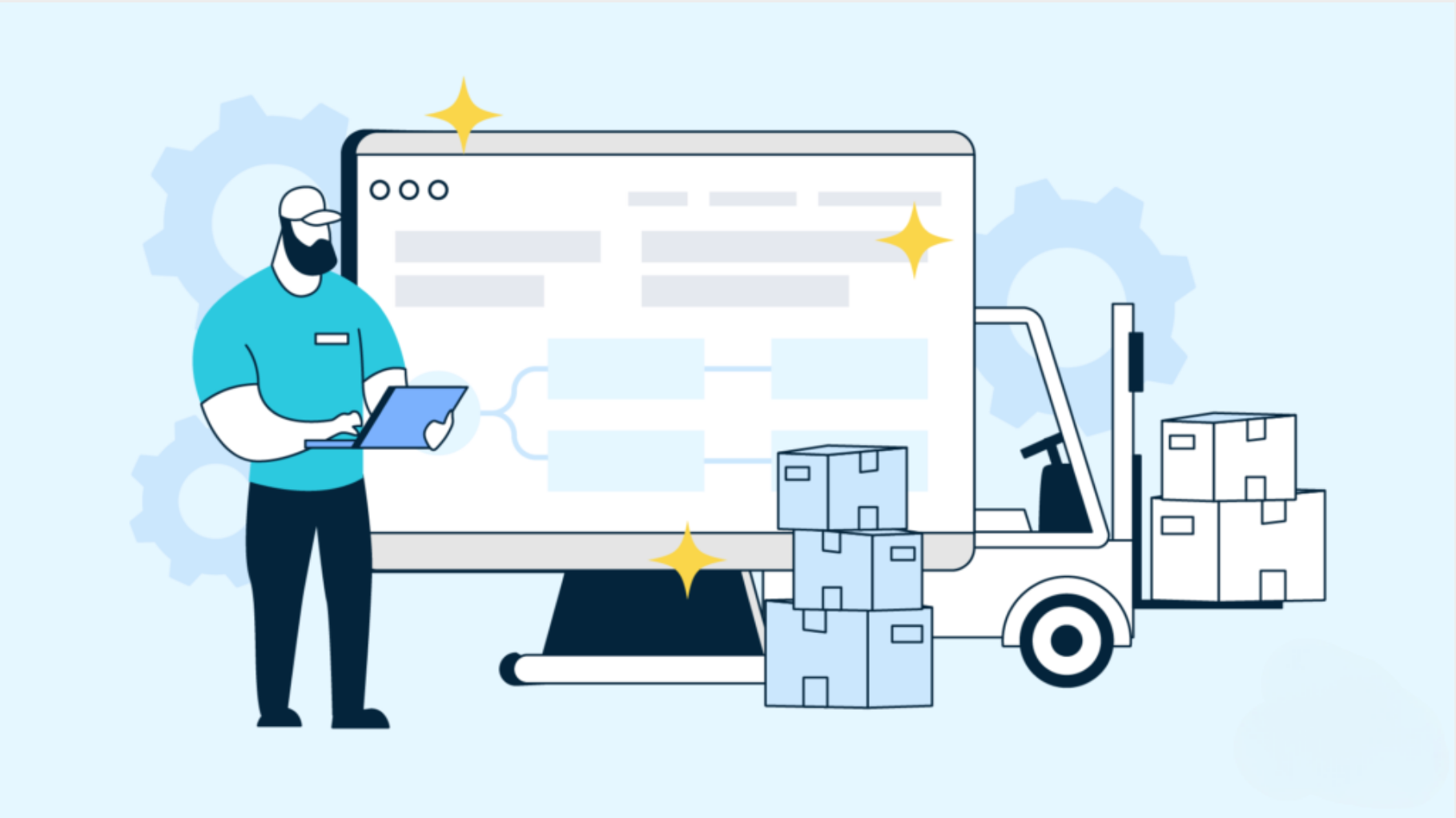
1. Demand Forecasting and Inventory Optimization
AI-powered predictive analytics processes historical sales data, market trends, and external variables like weather or economic conditions to predict demand accurately. Machine learning models refine these forecasts continuously, ensuring optimal inventory levels to minimize overstock and stockouts.
2. Route Optimization and Logistics Efficiency
Advanced AI algorithms calculate efficient delivery routes by analyzing traffic, weather, and delivery constraints. This reduces costs, minimizes delays, and enhances last-mile delivery performance.
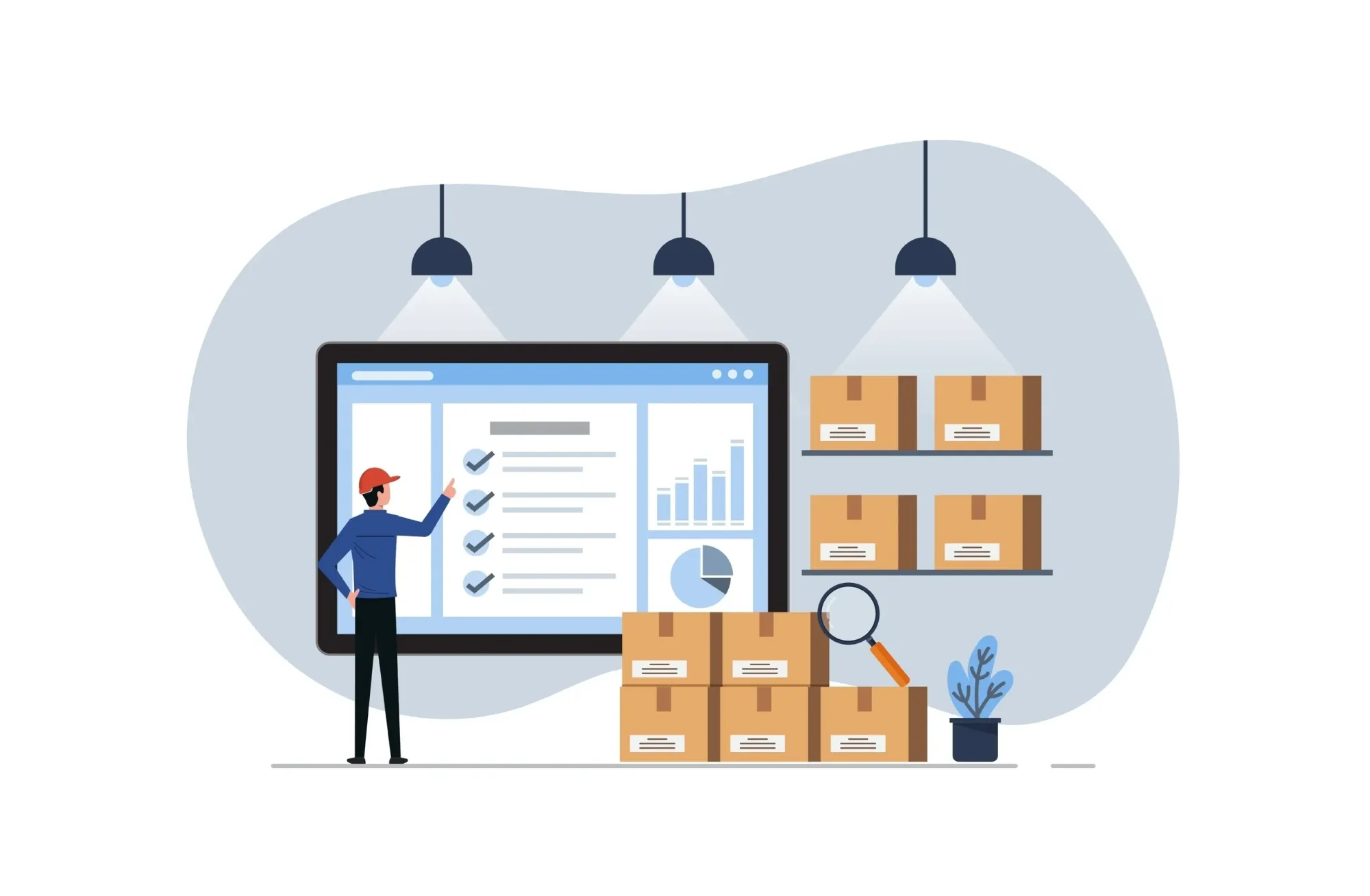
3. Personalized Retailer Engagement
AI can analyze retailer behavior and purchase history to generate personalized product recommendations, increasing sales through targeted promotions, cross-selling, and upselling.
4. Real-time Insights and Decision-making
AI-driven systems can process vast amounts of data in realtime, delivering actionable insights for quick decision-making, such as identifying slow-moving products or predicting stock shortages.
5. Fraud Detection and Prevention
AI’s anomaly detection capabilities identify unusual patterns in distributor claims or transactions, mitigating fraud risks and ensuring compliance.
6. Enhanced Workforce Productivity
AI-powered chatbots and automation tools handle repetitive tasks like order processing, allowing human resources to focus on strategic activities.
AI/ML Modules with Python Implementations
To demonstrate the integration of AI/ML in DMS, here are key modules with Python code examples:
1. Demand Forecasting Module
Predict future demand based on historical sales data using machine learning:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestRegressor
# Load sales data
data = pd.read_csv('sales_data.csv')
X = data[['product_id', 'region', 'season', 'promotions']]
y = data['sales']
# Train/test split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train model
model = RandomForestRegressor()
model.fit(X_train, y_train)
# Predict demand
predictions = model.predict(X_test)
2. Route Optimization Module
Calculate the optimal delivery route using Dijkstra’s algorithm:
import networkx as nx
# Define the graph
graph = nx.Graph()
graph.add_weighted_edges_from([
('Warehouse', 'Retailer1', 5),
('Warehouse', 'Retailer2', 7),
('Retailer1', 'Retailer2', 2),
('Retailer1', 'Retailer3', 3),
('Retailer2', 'Retailer3', 4),
])
# Find shortest route
shortest_path = nx.dijkstra_path(graph, source='Warehouse', target='Retailer3')
print(f"Optimal Route: {shortest_path}")
3. Retailer Recommendation Module
Recommend products to retailers using collaborative filtering:
from sklearn.neighbors import NearestNeighbors
import pandas as pd
# Retailer purchase data
data = pd.DataFrame({
'retailer_id': [1, 1, 2, 2, 3, 3],
'product_id': [101, 102, 101, 103, 102, 104],
'purchase_count': [5, 3, 6, 2, 4, 1]
})
# Create pivot table
pivot_table = data.pivot_table(index='retailer_id', columns='product_id', values='purchase_count', fill_value=0)
# Train model
model = NearestNeighbors(metric='cosine')
model.fit(pivot_table)
# Recommend products
retailer_id = 1
distances, indices = model.kneighbors([pivot_table.loc[retailer_id]])
print(f"Recommended Retailers: {indices[0][1:]}")
Key Challenges and Future Outlook
While the adoption of AI/ML in DMS is transformative, challenges like data quality, scalability, and change management persist. Implementing robust data governance, investing in scalable infrastructures, and fostering a culture of innovation are critical to overcoming these hurdles.
Looking ahead, trends such as autonomous supply chains, predictive maintenance, and hyper-personalization will drive the evolution of AI/ML in DMS, further transforming the CPG industry.
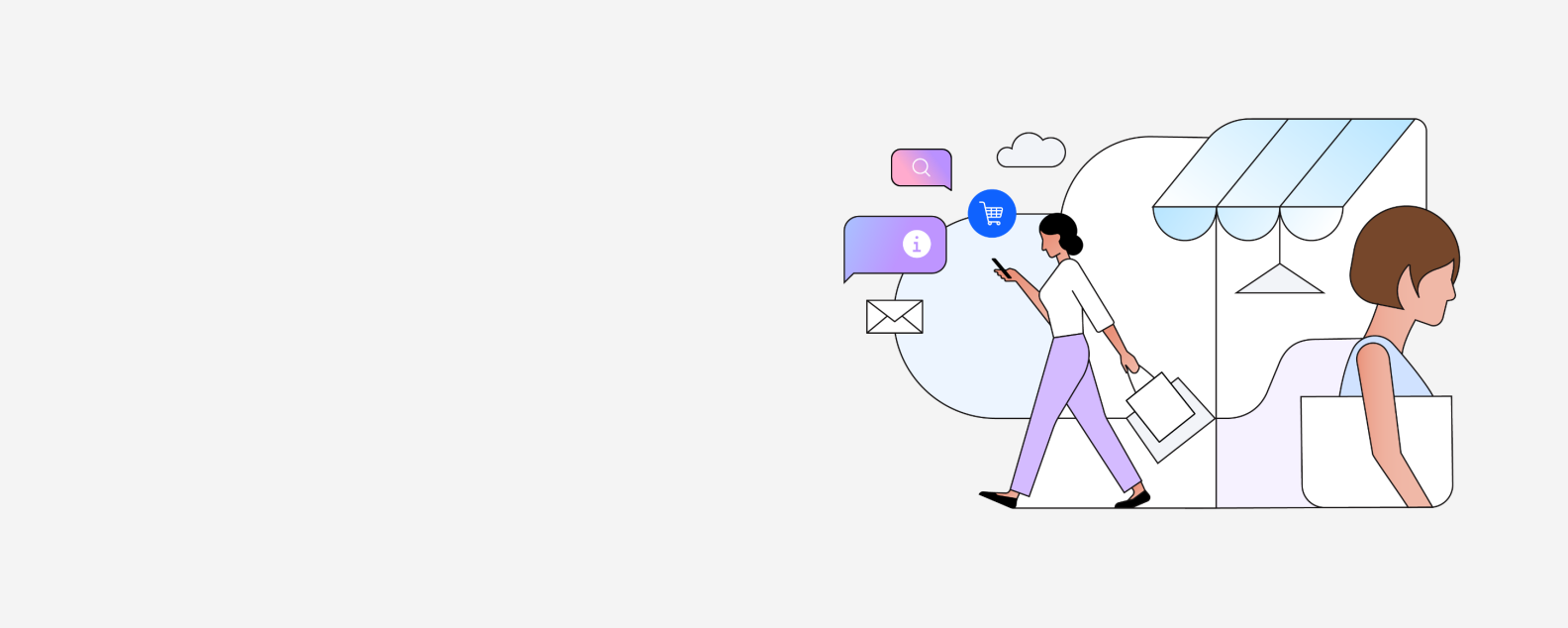